Leveraging API Routes in Next.js for Better Performance
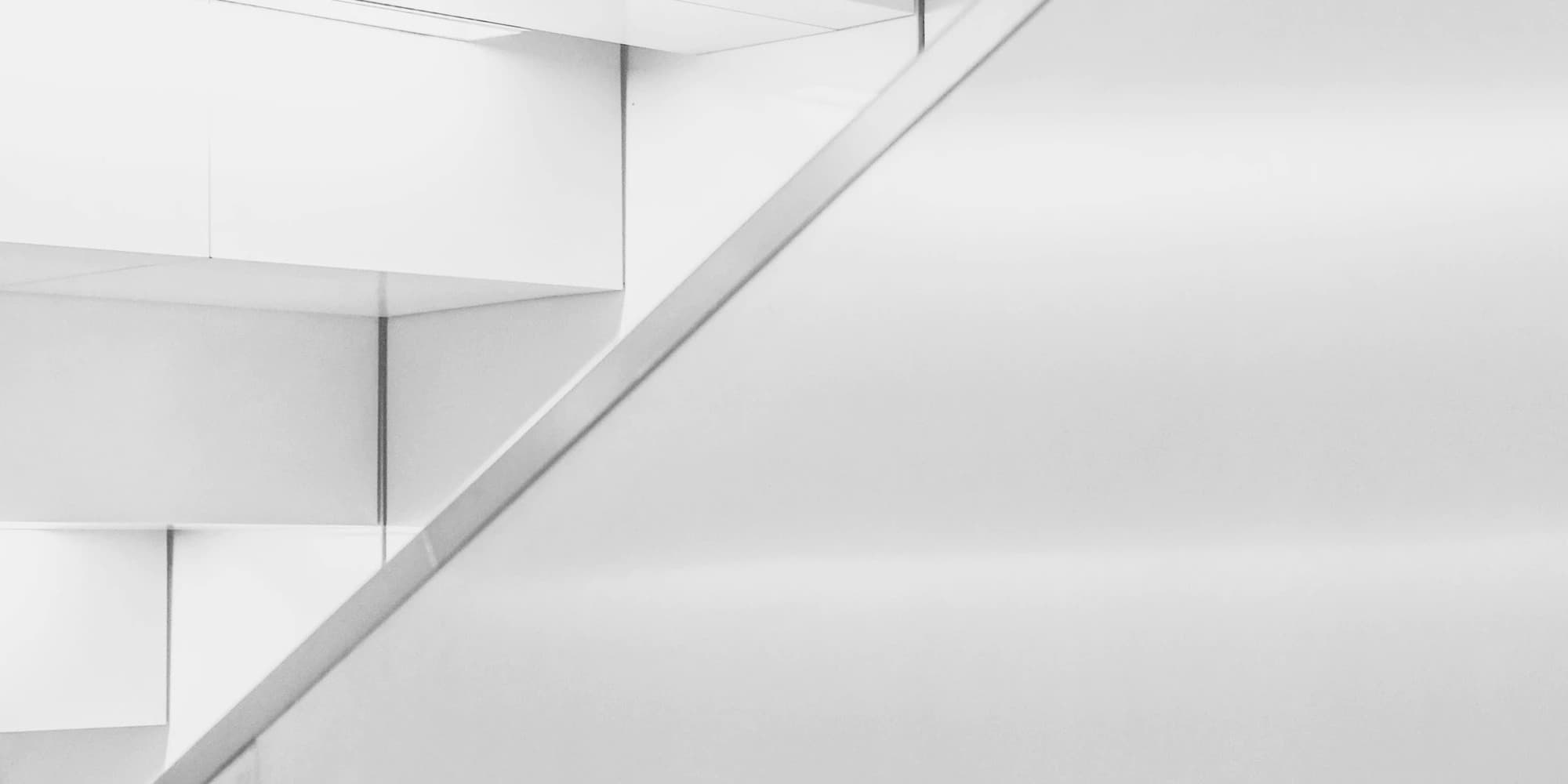
API routes in Next.js provide a powerful way to build serverless APIs that can be used to enhance the performance and functionality of your web applications. Whether you're fetching data, handling form submissions, or integrating third-party services, API routes make it easy to add backend functionality without the need for a separate server.
In this article, we'll explore how to create and use API routes in Next.js and discuss their benefits for modern web development.
What are API Routes?
API routes in Next.js allow you to create endpoints within your application that can be called from the frontend or other services. These routes are serverless functions that run on-demand, providing a scalable and efficient way to handle backend logic.
Benefits of API Routes:
- Serverless: No need to manage a server, reducing maintenance overhead.
- Scalable: Automatically scale based on demand, making them ideal for applications with varying traffic.
- Integrated: Seamlessly integrate with your Next.js application, sharing the same codebase and environment.
How to Create API Routes in Next.js
Creating an API route in Next.js is simple. Just add a file under the pages/api
directory, and it will automatically become an API endpoint. Here's an example:
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: "Hello, world!" });
}
In this example, the /api/hello endpoint returns a JSON response with a simple message.
Using API Routes in Your Application You can call your API routes from any part of your application using fetch or other HTTP request methods. For example:
useEffect(() => {
fetch("/api/hello")
.then((response) => response.json())
.then((data) => console.log(data.message));
}, []);
This code fetches data from the /api/hello route and logs the response to the console.
Conclusion
API routes in Next.js provide a flexible and powerful way to add backend functionality to your web applications. By leveraging these serverless endpoints, you can build more efficient and scalable applications without the complexity of managing a traditional server.